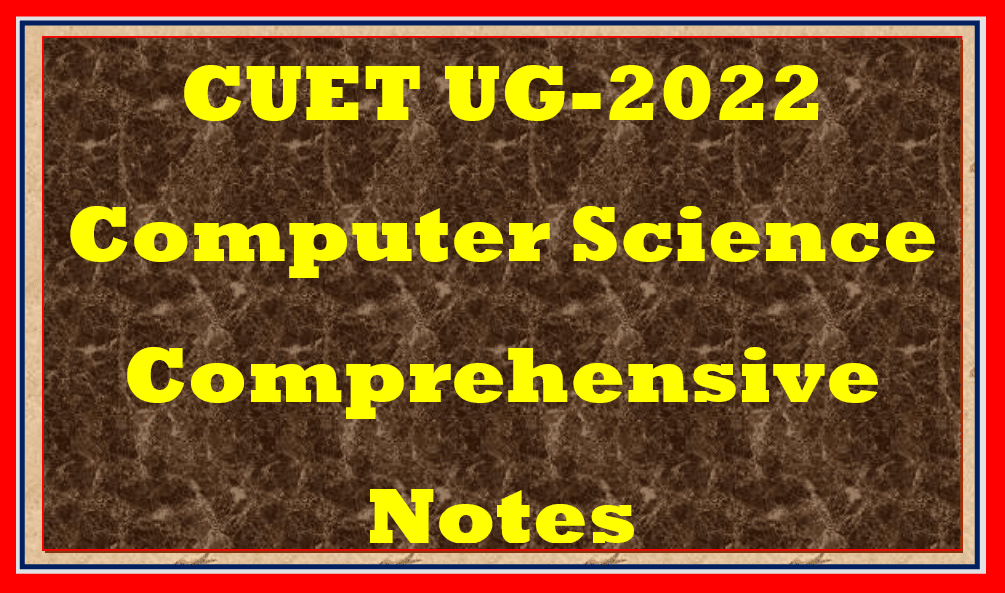
In this article, CUET Computer Science Informatics Practice 308 comprehensive notes I am going to discuss the content in detail. So here we go!
Topics Covered
CUET Computer Science Informatics Practices 308 Syllabus
I have already written one article for CUET Computer Science Informatics Practices 308 syllabus. Follow this link to download and understand the same.
Let us begin the article with Section A. Section A which is compulsory for all students. So here we go unit-wise notes for CUET Computer Science Informatics Practices 308. Let’s begin!
Section A
This section contains notes for CUET UG 2022 Computer Science Section A. From this section 15 questions will be asked which are compulsory for all candidates. Explore the contents now!
Exception handling in Python
In the first section of CUET Computer Science Informatics Practices 308 comprehensive notes, I am going to discuss the topic of exception handling. So let’s begin with the basics of exception handling!
What is an exception?
While compiling the programs sometimes the program will not return the desired output. It generates garbage output or the program behaves abnormally. This can happen due to syntax errors, runtime errors, or logical errors.
Before understanding exceptions you need to understand different types of errors.
Syntax Errors
Every programing language follows some rules and regulations for writing programs. When these rules are not followed while writing the program, syntax errors occur. It is also known as parsing errors.
In python when a syntax error occurs python will now allow going ahead in the program until errors are rectified. Python returns the Name of Error and a small description of the error. Observe the following screenshot.

So the syntax error is displayed in the interactive mode or python shell window.
If you are working in script mode it will return the error in the Error Dialog box with the title SyntaxError and description with the OK button. Observe the below-given screenshot.

The script mode won’t allow you to go ahead until this error is rectified in the program. The syntax error will be returned by python itself.
Runtime Errors
The program is syntactically correct but having issues that are detected during only the program execution is known as runtime errors. It cannot be detected by python itself.
To fix them you have to correct the code according to the program.
Logical Errors
The runtime errors which produce an incorrect output is known as logical errors. Sometimes logical errors cause the programs to crash.
Now let’s understand what is an exception?
“An exception is an event, which occurs during the execution of a program that disrupts the normal flow of the program’s instructions.”
— TutorialsPoint
An exception is a Python object that represents an error. When an error occurs during the execution of a program, an exception is said to have been raised.
– NCERT Text Book Computer Science Class 12
Remember some key points related to exceptions:
- Exception needs to be handled by a developer.
- The program does not terminate abnormally.
Built-in exceptions in python
Commonly occurring exceptions defined by the compiler/interpreter is known as a built-in exception.
Python standard library contains an extensive collection of built-in exceptions. When any of these exceptions occur in the program, the appropriate exception handler code is executed and displays the reason and name of that exception. The programmer has to take appropriate action to handle it.
Python supports some built-in exceptions which are as follows:
S.NO | Exception | Description |
1 | SyntaxError | Occurs when an error in python code |
2 | ValueError | Occurs when a function receives an argument with a mismatched or inappropriate value |
3 | IOError | Occurs when the file given in the statement can’t open |
4 | KeyboardInterrupt | Occurs when the normal flow of program is interrupted due to pressing any key such as delete or backspace |
5 | IndexError | Occurs when index or subscript of a list, tuple or any other object is out of range |
6 | IndentationError | Occurs when indentation is not maintained properly in the program |
7 | ImportError | When the imported module is not available this exception occurrs |
8 | EOFError | Occurs when End Of File condition is reached without reading any data |
9 | NameError | Occurs when local or global variable is not defined |
10 | TypeError | Occurs when the operator is given with a value of incorrect data type |
11 | OverFlow Error | Occurs when the calculation exceeds the maximum limit |
12 | ZeroDivisionError | Occurs when a divisor is zero |
User-Defined Exceptions
The user can also create their own exception in the program as per their requirements. These exceptions are called user-defined exceptions.
Python supports two kinds of statements to create user-defined exceptions. They are:
- raise
- assert
raise statement
It is used to throw an exception. The syntax of the raise statement is as follows:
raise exception-name[(option argument)]
Example:
pwd = 123
if type(pwd)!=str:
raise TypeError("Password must be a strig not a number")
Output:

The assert statement
It is used to test an expression in the python program. If it returns the result False then the exception is raised. This is generally used at the beginning of the function or a function call to check for valid input.
Syntax:
assert Expression [,arguments]
Example:
x =int(input("Enter the no."))
assert x%2==0
print('x is even no.')
Output:

In the above code, the assert statement evaluates the value for a given number. If it is even then it prints the message “x is even no.” when it evaluates False then it raises AssertionError.
Handling Exceptions
When the user writes an appropriate code in a program to display proper messages or instructions to the user when an exception is raised is called handling exceptions.
Need for exception handling
The need for exception handling is very crucial for programs to avoid abnormal crashing of the program. Following are some important points regarding exceptions and their handling:
- There are different types of exceptions in python. So to handle the different exception handler code needs to be written.
- Exception handlers have these parts in the code:
- The main logic of the program for error detection and error correction
- The segment of code which detects an error placed inside one block
- The execution code placed inside another block
- The compiler or interpreter keeps track of the exact position where an error has occurred
- Exception handling is needed for built-in and user-defined functions
How to handle exceptions in Python
Python automatically creates an exception object when an error occurred in the program. This object has information such as:
- error type
- file name
- position in the program
This object is passed on to the run time system to find a suitable code to handle the exception. This process is called throwing an exception.
Note: When an exception occurs in the program the control jumps to an exception handler and stops the execution of the program.
Exception handler refers to a block of code which handle raised exception. It will search the following:
- A method in which error is detected
- If the error method is not found it will search in the function called
This exception handling is going to execute the code in hierarchical search is called a stack.
When it founds the suitable handler is found in the call stack it is executed by the run time process. This process is known as catching the exception.
If the stack is unable to find a suitable exception then it stops the program. The entire process is explained in the following diagram:

Catching exception
To catch exceptions try and except blocks are used. An exception is said to be caught when a code that is designed to handle a particular exception is executed.
The try block contains the lines of codes where exceptions might occur. The except block followed by the exception name contains the code to handle the exception when the error orrucrs.
Syntax:
try:
code where exception might occur
except [exception-name]:
exception handling code
Example:
try:
print(n)
except NameError:
print("Variable n not defined")
Output:

In the above code, n is printed without declaring a variable so it raises NameError which is caught in except in block.
Handling multiple exceptions
Multiple exceptions can be caught by using except block multiple times.
Example:
n="Hello"
try:
print(n+5)
except NameError:
print("Variable n not defined")
except:
print("n should be integer")
Output:

try-except-else-clause
The try-except-else block is used to handle the exception in the case when there is no known error or exception and none of them except block is executed else clause will be executed.
Example:
n=10
try:
print(n)
except NameError:
print("Variable n not defined")
except:
print("Somthing went wrong")
else:
print("No error")
Ouptut:

Finally Clause
The finally clause is the final clause of exception handling. It is common practice to use finally clause while working with files to close the file object.
The finally clause must be placed at the end of the exception handling code after all except blocks and else block.
n=10
try:
print(n)
except NameError:
print("Variable n not defined")
except:
print("Somthing went wrong")
else:
print("No error")
finally:
print("The END")

recovering and counting in finally
If an error has been detected in the try block and the exception has been thrown, the appropriate except block will be executed to handle the error. But if the exception is not handled by any of the except lauses,
then it is re-raised after the execution of the finally block.
If any other type of error occurs for which there is no handler code (except clause) defined, then also the finally clause will be executed first.
print (" Practicing for try block")
try:
numerator=50
denom=int(input("Enter the denominator"))
quotient=(numerator/denom)
print ("Division performed successfully")
except ZeroDivisionError:
print ("Denominator as ZERO is not allowed")
else:
print ("The result of division operation is ", quotient)
finally:
print ("OVER AND OUT")
While executing the above code, if we enter a non-numeric data as input, the finally block will be executed. So, the message “OVER AND OUT” will be displayed. Thereafter the exception for which handler is not present will be re-raised.
After execution of finally block, Python transfers the control to a previously entered try or to the next higher level default exception handler.
In such a case, the statements following the finally block is executed. That is, unlike except, execution of the finally clause does not terminate the exception. Rather, the exception continues to be raised after execution of finally.
File handling – CUET Computer Science Informatics Practices 308
Follow the beloe given links for the content on file handling:
- Introduction to File handling & Working with Text Files in pyhton
- Working with binary files in python
- Operations on binary files
- Working with CSV files
Database Concepts and Structure Query Language – CUET UG 2022 Computer Science 308
Follow this link for Database Concepts and Structure Query Language – CUET UG 2022 Computer Science 308:
Computer Networks – CUET UG 2022 Computer Science 308
- Introduction to computer networks and evolution of networking
- Communication Media
- Network Types & Topologies
- Network Devices
- Basics of web site and internet
Section B1: Computer Science
In this section you will get notes for Section B1: Computer Science for CUET UG 2022 Computer Science. From this section 35 questions will be asked out of which 25 questions need to be attempted. Explore the notes from the below given links:
Chapter 1: Exception and File Handling in Python
We have already covered this topic in Section A. Refer to Section A for notes on this topic.
Chapter 2: Stack
Introduction to Data Structure Stack and Implementation using Lists
The remaining chapters will be updated soon.
Chapter 7 : Database Concepts
We have already covered these topics in section A. Refer to section A.
Chapter 8 : Structured Query Language
We have already covered these topics in section A. Refer to section A.
Chapter 9: Computer Networks
We have already covered these topics in section A. Refer to section A.
Section B2: Informatics Practices
CUET UG 2022 Syllabus Section B2 contains the topics from Informatics Practices. From this section 35 questions will be asked out of this 25 questions need to be attempted.
Follow this link for all chapters of Section B2: Informatics Practices Class 12.